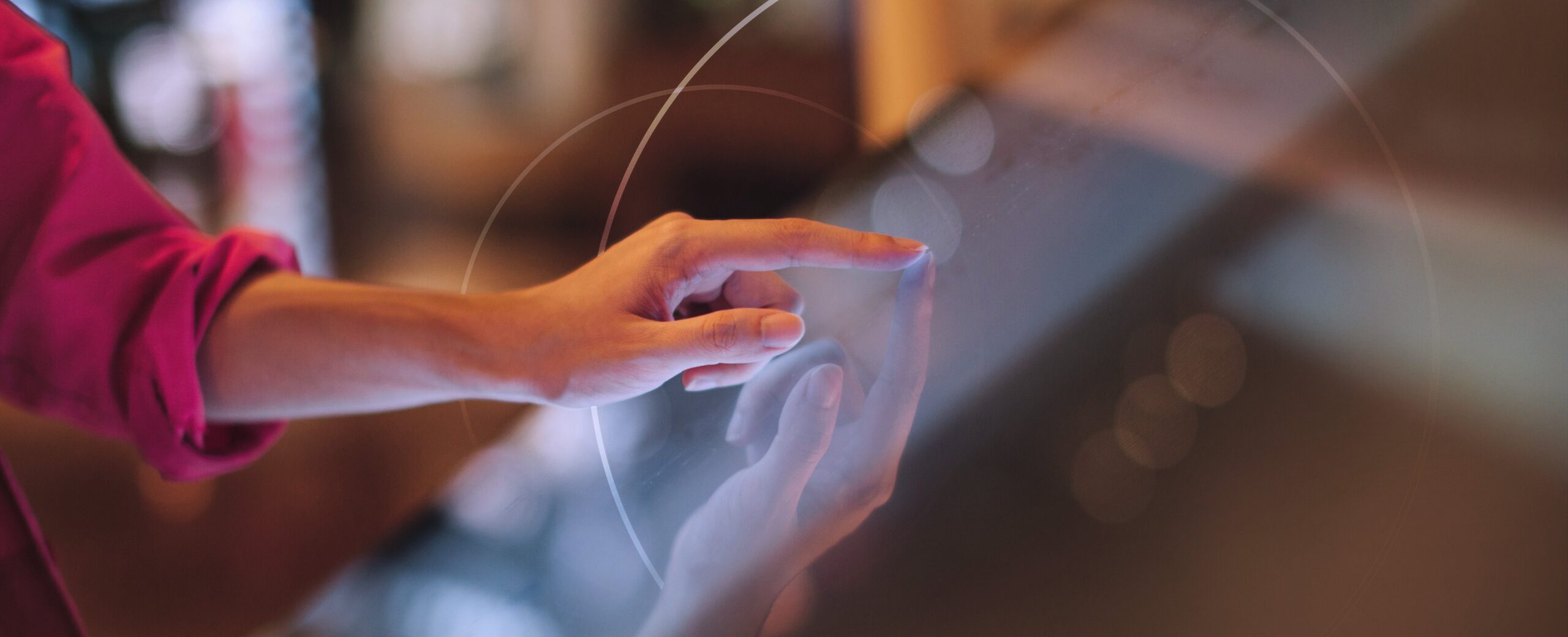
The front-end is the client-side part of your application.
Everything that is visible to your users is part of the front-end.
To put it in other words — front-end testing is about graphical user interface (GUI) testing.
So you get a better picture, if you are developing an online shopping platform, frontend testers check whether the look and feel of the website are aligned with the requirements. Also, they make sure things work properly and feel the way the user experience designer envisioned it.
Why is front-end testing so important?
Well… because our end users judge the book by its cover and have no clue about the back-end side of things, they only notice when there’s some issue they can see. Hence problems in the UI stick out and get noticed immediately. A company that wants to be successful thus needs to provide a great and consistent user experience. This means making sure that the application runs fast and without error, no matter which device or browser is used.
What is automated front-end testing?
Probably you are already familiar with manual testing. For example, you have implemented an input field into a form. Afterwards, you open the webpage click into the input field to check whether it works and submit the form. This was a manual test. Performing this test for everything you implement over and over again is tiresome and not scalable. At a certain point, you would have no time to implement anything anymore and would spend all day testing. This is where automatization comes into play. Using automated concepts we can be sure that features will continue to work as they should.
Automated tests also help especially with so-called edge cases. Use cases we tend to forget, like checking a box for some signup process.
What are the top reasons to automate testing?
- Update your code with confidence! Good tests allow you to refactor code with confidence that you are not breaking any functionality and without changing the tests.
- Documentation included, for FREE! Tests come in handy because they explain how your code works with its expected behaviour. Tests, not like written documentation often, have to be always up to date.
- Bugs are gone forever — almost! By writing up test cases for every bug, you can be sure that these will never come back. Further writing tests will improve your understanding of the code and the requirements. Using testing you might find issues that you could miss otherwise.
What is being tested in front-end testing?
UNIT TESTS
These are focused on the smallest entity of your front-end applications.
Usually, these are components in the React context, but cover interfaces, classes and methods. These simple and small tests check whether the output of a small part equals the expected. The checks are predefined and completely isolated.
INTEGRATION TESTS
Like the name suggests, these type of tests put multiple code components together and test their interaction. In React these are usually built of views that integrate multiple components.
END-TO-END TESTS
These tests are the holy grail since they are testing the application. Let’s dive in a littler further on end-to-end testing.
What is end-to-end testing?
End-to-end testing or user interface testing is an approach to testing a web application. An end-to-end test verifies that a web application meets the requirements and works as expected. The so-called “user flow” is tested. End-to-end testing is important! But nobody likes it…They can be extremely cumbersome and very time-consuming. On the other hand, only testing can provide confidence in the final product. In the end, testing ensures that we don’t release a broken product to our users.
What is Cypress?
Cypress is a free, open-source automation testing tool built for modern web applications based on modern frameworks like React. It provides a UI to show what tests and commands are running, passing and/or failing.
But isn’t there Selenium?
Every developer has suffered before configuring Selenium. There are a multitude of steps, often extremely confusing, where we need compatible versions of Java, drivers, browsers, even Selenium itself, etc… It’s just exhausting! Not to mention that many front-end developers don’t necessarily want to use Java. Additionally, Cypress easily outperforms Selenium in terms of speed, reliability, and ease of use.
Cypress Installation & Configuration
Cypress comes with some documentation dedicated to visual testing and a list of all available plug-ins to help configure it for visual regression testing.
We decided to choose cypress-plugin-snapshots because of the ease of use and amount of time needed for the initial setup. There are many great tools & libraries out there, so check them out!
We simply installed the npm library like so:
npm install cypress cypress-plugin-snapshots
And this is how a cypress.json configuration can look:
{ “env”: { “TAGS”: “not @ignore”, “cypress-plugin-snapshots”: { “autoCleanUp”: true, “autopassNewSnapshots”: true, “diffLines”: 3, “prettier”: true, “imageConfig”: { “createDiffImage”: true, “resizeDevicePixelRatio”: false, “threshold”: 0.025, “thresholdType”: “percent” }, “screenshotConfig”: { “blackout”: [“”], “capture”: “viewport”, “disableTimersAndAnimations”: true, “log”: true, “scale”: false, “timeout”: 30000 } } }, “baseUrl”: “http://localhost:9000”, “video”: false, “viewportWidth”: 1920, “viewportHeight”: 1080, “testFiles”: “**/*.feature”, “requestTimeout”: 60000, “ignoreTestFiles”: [“**/__snapshots__/*”, “**/__image_snapshots__/*”], “chromeWebSecurity”: false, }
Why integrate Cucumber with Cypress?
There are several tools for a behavior-driven development framework, but the most commonly used BDD tool is Cucumber. BDD is a form of Test Driven Development (TDD). Human-readable descriptions of software requirements are used as the basis for tests. This approach helps us to write the tests in a common vocabulary for all project participants. For this reason, even someone without a developer background can get an idea of the tests carried out.
Cucumber with Gherkin
For Cypress we can only write our tests in Javascript /TypeScript. However, if we want to use Cucumber, we convert the Java Script test to “Gherkin” (the language for Cucumber). Gherkin mainly consists of the four main keywords: “Given”, “When”, “And” and “Then”. Using the keywords we can use the already used JavaScript code.
JavaScript end-to-end tests in Cypress
{ it(“Can fill out the form”, () => { cy.visit(“/”); cy.get(“form”); cy.get(‘input[name=”name”]’) .type(“David”) .should(“have.value”, “David”); cy.get(‘input[name=”email”]’) .type(“david@devoteam.com”) .should(“have.value”, “david@devoteam.com”); cy.get(“textarea”) .type(“This is a test”) .should(“have.value”, “This is a test”); cy.get(“form”).submit(); }); });
With just one look you can see that it will be very difficult for someone without developer knowledge to understand the pure test script.
The same with Cucumber and Gherkin
//integration/formular/form.ts import { Before, Given, Then, When, And } from ‘cypress-cucumber-preprocessor/steps’; Given(“We visit the form page”, ()=>{ cy.visit(“/”); cy.get(“form”); }); When(“we enter the name {String}”, (name) => { cy.get(‘input[name=”name”]’) .type(name) .should(“have.value”, name); }); And(“we enter the email {String}”, (email) => { cy.get(‘input[name=”email”]’) .type(email) .should(“have.value”, email); }); And(“we enter the comment {String}”, (comment) => { cy.get(‘textarea’) .type(comment) .should(“have.value”, comment); }); And(“we submit the form”, () => { cy.get(“form”).submit(); }); Then(“we are on the home page”, () => { cy.url().should(“include”,”start”); });
The .feature test file
Functionality: Testing the form input Scenario: F-01 Enter the form and submit Given we visit the form page When we enter the name “David” And we enter the email “david@devoteam.com” And we enter the comment “This is a Test” And we submit the form Then we are on the home page
//integration/form.feature
#language: en
Functionality: Testing the form input
Scenario: F-01 Enter the form and submit
Given we visit the form page
When we enter the name “David”
And we enter the email “david@devoteam.com”
And we enter the comment “This is a Test”
And we submit the form
Then we are on the home page
However, if we now look at the test file with Cucumber, it becomes much simpler and more readable.
Some final words
Cypress with Cucumber is a great test framework and in my opinion, has the following advantages:
- Easy to install and configure
- Faster by running the tests directly in the application
- Has a powerful real-time debugger
- Easy to write because JavaScript — no need for Java
- Useful and simple API
- Super detailed documentation
- Very active community
How can I learn more?
This article is a part of a greater series centred around the technologies and themes found within the first edition of the Devoteam TechRadar. To read further into these topics, please download the TechRadar.
u003ch3u003eu003cstrongu003eWant to know more about Cypress?u003c/strongu003eu003c/h3u003ernCheck out our TechRadar to see what our experts say about its viability in the market.
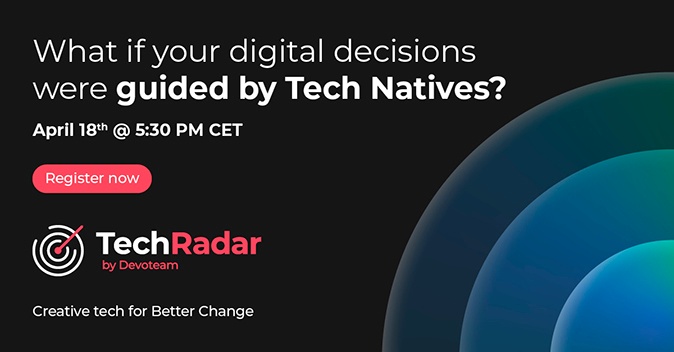